What are Gangs of Four Design Patterns in Software Development: A Comprehensive Guide
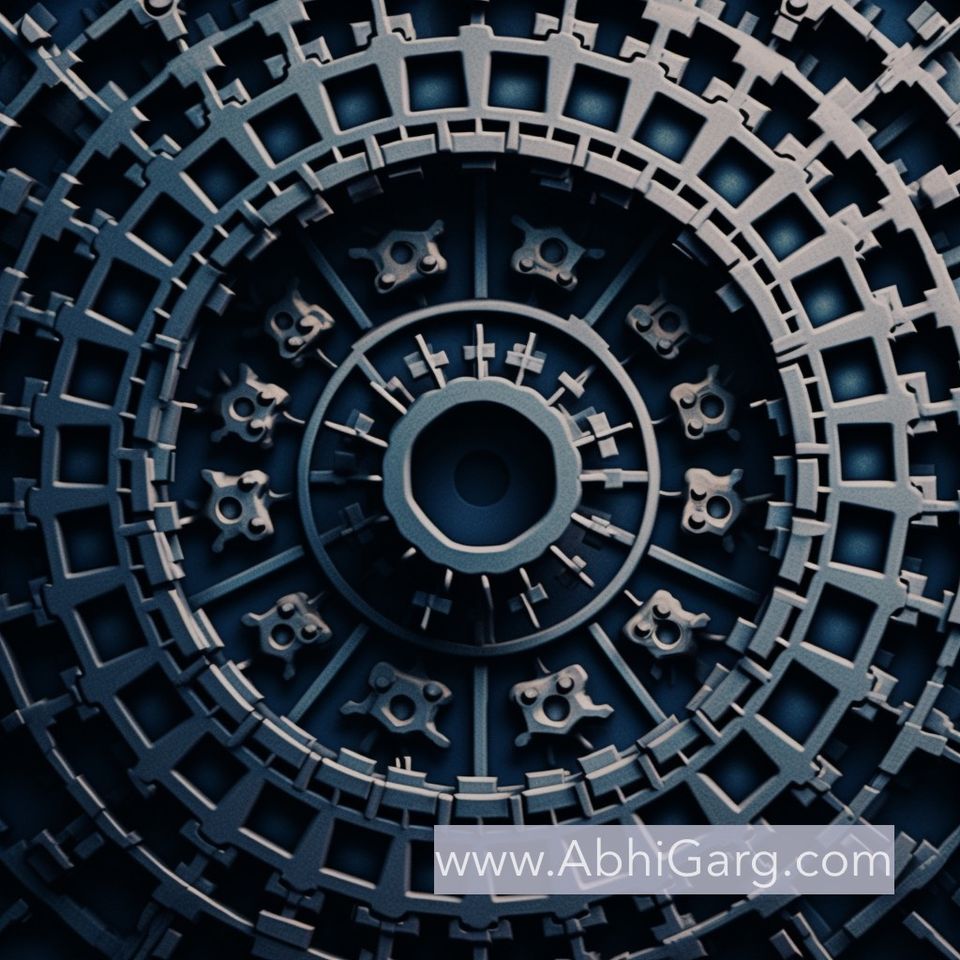
Hello there, brave souls of the software world! Today, we're delving into the deep, occasionally murky, but always enlightening world of design patterns. Like a trusty old map, these patterns guide us through the daily challenges we, the software engineers, face. So sit tight, grab a cup of coffee—or tea if you're a rebel—and let's navigate through these gems together.
What Are Design Patterns?
Before diving into the nitty-gritty, let's quickly understand design patterns. Think of them as reusable templates to solve problems. You've got a problem; they've got a pattern—a match made in heaven, or at least in code.
What are the different Gangs of Four (GoF) Design Patterns in Software Development?
The Gangs of Four Design Patterns are broken into:
- Behavior
- Structural
- Creational
Then, there are Cloud Design Patterns—more on the Cloud angle in another post.
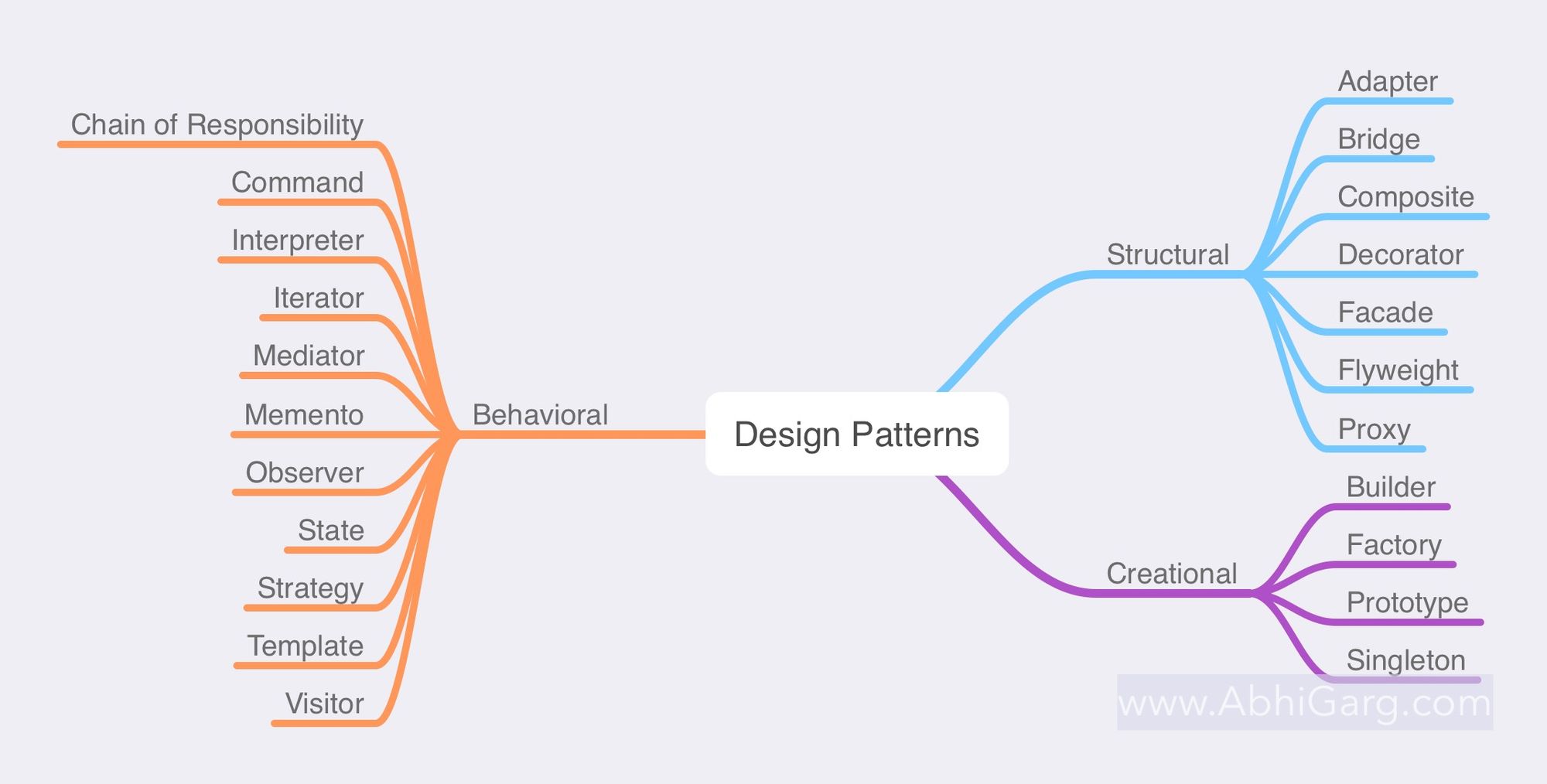
Behavioral Design Patterns
Ah, behavior—the one thing we wish we could easily change in our pets, kids, and occasionally ourselves. Behavioral patterns deal with the responsibilities and communication between objects.
Chain of Responsibility
What It Is: This pattern passes a request along a chain of handlers. Each handler either processes the request or passes it along the chain.
Example: Consider customer support, where your query may pass from a bot to a first-tier representative and, perhaps, to a specialized agent.
Command
What It Is: It turns a request into an independent object containing information about the request.
Example: In GUI libraries, each action, like a button click, can be a command object.
Interpreter
What It Is: Provides a way to evaluate expressions in a language.
Example: Parsing XML files or SQL queries.
Iterator
What It Is: It provides a way to traverse elements of a collection without exposing its underlying implementation.
Example: Think of browsing through your playlist without worrying about how the songs are stored.
Mediator
What It Is: An object that encapsulates how objects interact.
Example: Air traffic control room, mediating between pilots.
Memento
What It Is: It captures an object's internal state to be restored later.
Example: Think of an undo feature in text editors.
Observer
What It Is: Defines a one-to-many relationship so that all its dependents are notified when one object changes state.
Example: Event handling systems.
State
What It Is: Allows an object to alter its behavior when its internal state changes.
Example: Think of a vending machine that behaves differently based on its stock.
Strategy
What It Is: Defines a family of algorithms and makes them interchangeable.
Example: Different compression algorithms like ZIP or RAR.
Template
What It Is: Defines a skeleton of an algorithm and lets subclasses redefine specific steps.
Example: Different types of coffees, same brewing process.
Visitor
What It Is: It lets you add further operations to objects without modifying their classes.
Example: Adding a print feature to different shape classes.
Structural Design Patterns
These guys are like the architects of the software world, focusing on how objects are composed or how classes inherit from each other.
Adapter
What It Is: It allows two incompatible interfaces to work together.
Example: Using a USB-C to USB-A adapter for your computer.
Bridge
What It Is: Separates abstraction from implementation so they can vary independently.
Example: Separating UI and business logic.
Composite
What It Is: It lets you compose objects into tree structures representing part-whole hierarchies.
Example: Graphic systems where shapes like circles and rectangles can be combined to create complex diagrams.
Decorator
What It Is: It adds new functionalities to an object without altering its structure.
Example: Adding scrollbars to a window in a graphical UI.
Facade
What It Is: It provides a simplified interface to a set of interfaces in a subsystem.
Example: Think of a home theater system with a single remote control.
Flyweight
What It Is: It minimizes memory usage or computational expenses by sharing as much as possible with related objects.
Example: Text editors that reuse character objects instead of creating a new one for each letter.
Proxy
What It Is: It provides a placeholder for another object to control access to it.
Example: Image proxy that delays loading of the actual image until needed.
Creational Design Patterns
Here, we focus on object-creation mechanisms.
Builder
What It Is: It lets you construct complex objects step by step.
Example: Building a meal at a fast-food restaurant.
Factory
What It Is: It provides an interface for creating objects without specifying the concrete class.
Example: Creating different types of accounts in a banking application.
Prototype
What It Is: Creates new objects by copying an existing object, known as the "prototype."
Example: Cloning DNA.
Singleton
What It Is: Ensures that a class has only one instance and provides a global point to access it.
Example: Database connections.
Closing Thoughts
In software development, design patterns are like the spices in your spice rack. You don't need them for every dish, but they can make a difference when you do.
Understanding when and how to use these patterns can separate the good developers from the great ones.
Remember, these patterns aren't a one-size-fits-all template but are more like guidelines to solve a problem in a proven and efficient way. So, use them wisely, adapt them as needed, and keep coding!
Happy Designing! 🎉